Bash Scripting: if elif else Statement (Complete Guide)

Introduction
Bash scripting serves as a powerful tool that enables developers to automate complex tasks & processes. One of the fundamental components of Bash scripting is the “if elif else” statement. This statement is used to implement particular code blocks based on specific conditions. In this comprehensive guide, you will gain insights into the various aspects of employing “if elif else” statements in Bash scripting.
You will learn the basics of conditional statements, including how to check for equality, inequality, and other logical operators. You will also get the highlights on different types of conditional statements available in Bash scripting, including the bash if statement, the “elif” statement, and the “else” statement. We have demonstrated how to use nested if statements and logical operators for creating more complex conditions.
Throughout this guide, we have given real-world examples and use cases to help you comprehend how to apply these concepts in your own Bash scripts. Whether you are a novice or a professional Bash developer, this guide provides you with the knowledge and skills needed to master the “if elif else” statement in Bash scripting. So, let’s begin and dive into the globe of Bash scripting and conditional statements.
Also Read: Bash Function: What is it and How to Use it? {Variables, Arguments, Return}
What is the Bash if Statement?
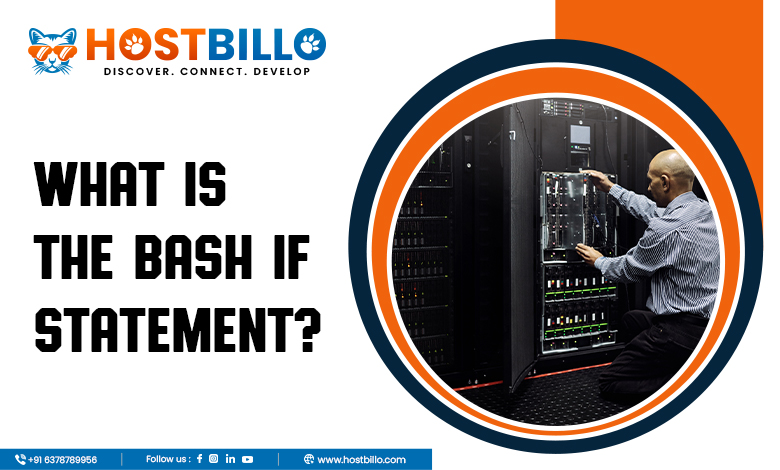
The Linux Bash if statement is a fundamental component of Bash scripting. It allows developers to execute specific code blocks based on certain conditions. It is a conditional statement that evaluates a Boolean expression and executes a set of statements if the expression is true.
In Bash scripting, the if statement can be used for a variety of tasks, such as checking the existence of a file or directory, verifying the success of a command, and comparing variables or strings. In addition to this, the if statement can be employed in conjunction with other conditional statements, such as the elif and else statements, to create more complex conditions.
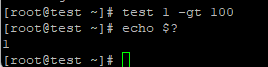

Bash if Statement Example
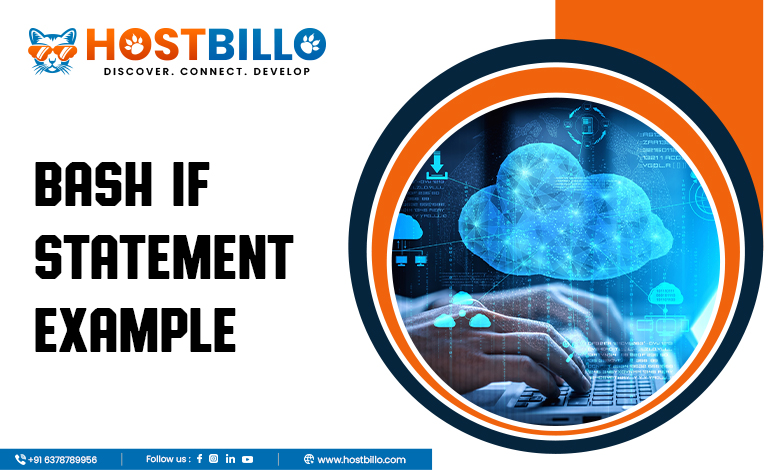
Here is an example of how the Bash if statement can be utilized in a script:
#!/bin/bash
read -p "Enter a number: " num
if [ $num -gt 0 ]; then
echo "The number is positive."
elif [ $num -lt 0 ]; then
echo "The number is negative."
else
echo "The number is zero."
fi
In this example, the script prompts the user to enter a number and then uses the if statement to check whether the number is positive, negative, or zero. If the number is greater than zero, the script outputs “The number is positive.” If the number is less than zero, the script outputs “The number is negative.” If the number is equal to zero, the script outputs “The number is zero.”
The if statement in this example uses the “-gt” and “-lt” operators to compare the value of the “num” variable to zero. If the expression evaluates to true, the corresponding statement(s) are executed.
This example illustrates how the Bash if statement can be employed to selectively execute code blocks based on conditions. By using the if statement, Bash developers can create scripts that automate complex tasks and processes by taking different actions based on the conditions present in the script.
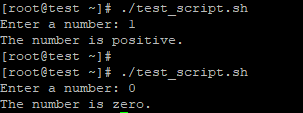
Bash if Statement Syntax
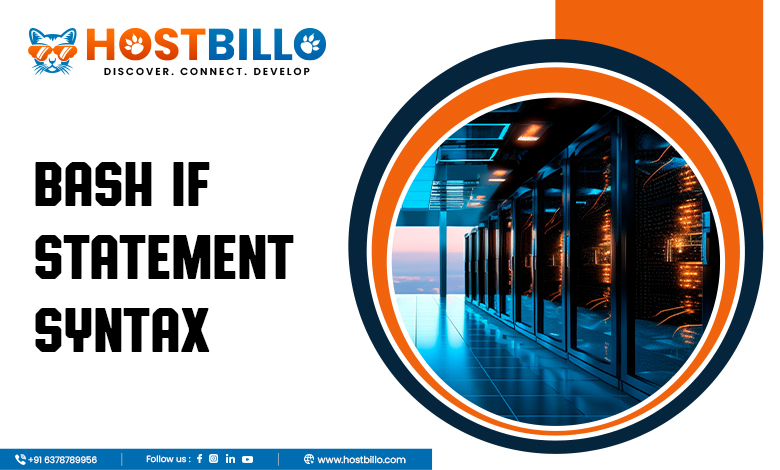
The Bash if statement is a conditional statement that enables developers to execute specific code blocks based on certain conditions. The syntax of the Bash if statement is as follows:
if [ expression ]; then
statement(s)
fi
The “expression” in the if statement can be any valid Bash expression that evaluates to true or false. The expression can include variables, constants, and operators that compare values or perform logical operations. The “statement(s)” that follows the if statement is executed only if the expression evaluates to true.
The “then” keyword is used to indicate the start of the code block that should be executed if the expression is true. The code block can consist of one or more statements, and it can contain any valid Bash commands or functions.
The “if” keyword is used to indicate the end of the code block linked with the bash if statement else. If additional conditions need to be checked, the if statement can be followed by one or more elif or else statements.
Furthermore, the Linux Bash if statement can be written using a variety of syntax options, including single parentheses, double parentheses, single-bracket, and double-bracket syntax. These syntax options provide developers with flexibility in how they write Bash conditional statements. They can easily choose the syntax that best fits their programming style and the requirements of their script.
Single-Parentheses Syntax
The single-parenthesis syntax is used to group and evaluate expressions. The syntax of the if statement using single parentheses is as follows:
if ( expression ); then
statement(s)
Fi
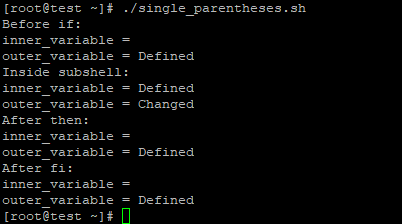
In this syntax, the expression is enclosed within a single set of parentheses, and the “then” keyword is followed by the code block that should be executed if the expression evaluates to true.
Double-Parentheses Syntax
The double-parenthesis syntax is used for arithmetic expressions. The syntax of the if statement using double parentheses is as follows:
if (( expression )); then
statement(s)
Fi
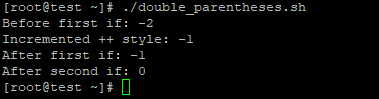
In this syntax, the expression is enclosed within a double set of parentheses, and the “then” keyword is followed by the code block that should be executed if the expression evaluates to true.
Single-Bracket Syntax
The single-bracket syntax is used for conditional expressions. The syntax of the if statement using single brackets is as follows:
if [ expression ]; then
statement(s)
fi
In this syntax, the expression is enclosed within a single set of brackets, and the “then” keyword is followed by the code block that should be executed if the expression evaluates to true.
Double-Bracket Syntax
The double-bracket syntax is used for extended conditional expressions. The syntax of the if statement using double brackets is as follows:
if [[ expression ]]; then
statement(s)
Fi
In this syntax, the expression is enclosed within a double set of brackets, and the “then” keyword is followed by the code block that should be executed if the expression evaluates to true.
Other Types of Bash Conditional Statements
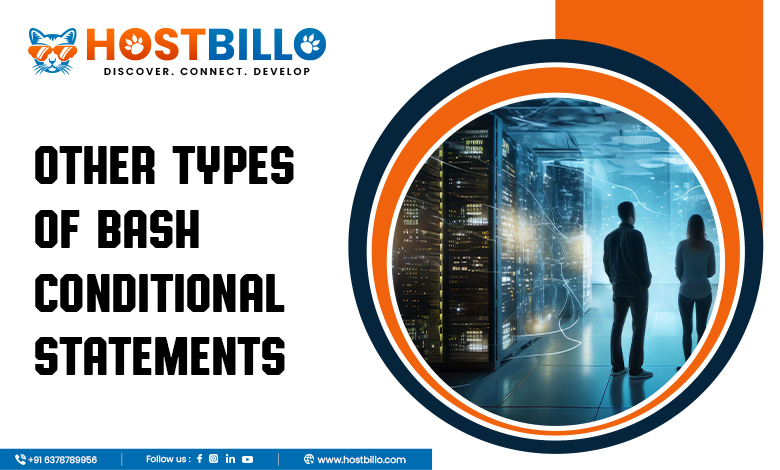
In addition to the basic if statement, several other types of Bash conditional statements can be employed to create more complex scripts. These include the if-else statement, if-elif statement, and nested if statement.
Bash if else Statement
The if-else statement permits developers to specify an alternate code block to execute if the expression in the if statement evaluates to false. The syntax of the if-else statement is as follows:
if [ expression ]; then
statement(s)
else
statement(s)
fi
In this statement, if the expression is true, the code block following the “then” keyword is executed. Otherwise, the code block following the “else” keyword is executed.
if elif Statement
The if elif statement is used to check multiple conditions in a script. The syntax of the if elif statement is as follows:
if [ expression1 ]; then
statement(s)
elif [ expression2 ]; then
statement(s)
else
statement(s)
fi
In this statement, if the expression1 is true, the code block following the “then” keyword is executed. If expression1 is false and expression2 is true, the code block following the “elif” keyword is executed. Otherwise, the code block following the “else” keyword is executed.
Nested if Statement
The nested if statement is used when one if statement is placed inside another if statement. This can be useful for checking complex conditions or creating more sophisticated logical structures. The syntax of the nested if statement is as follows:
if [ expression1 ]; then
if [ expression2 ]; then
statement(s)
fi
fi
In this statement, if expression1 is true, the inner if statement is evaluated. If expression2 is true, the code block following the “then” keyword is executed. If expression 2 is false, no action is taken.
Also Read: A Complete Guide to useradd Command in Linux with Examples
Conclusion
By now you must have acquired a comprehensive understanding of the “if elif else” statement in Bash scripting. Conditional statements are an assertive tool in automating complex tasks and processes, and mastering the “if elif else” statement is a fundamental step in becoming a proficient Bash scripter. Throughout this guide, we have covered the basics of conditional statements, including logical operators and different types of conditions. We have also explored more advanced concepts, such as nested if statements and logical operators for designing complex conditions. By observing real-world examples & use cases, you must have learned how to apply these concepts in your own Bash scripts. Moreover, mastering the “if elif else” statement will help you take your Bash scripting skills to the next level. We encourage you to experiment with the concepts and examples provided in this guide to obtain success in your Bash scripting endeavors!