Bash Function: What is it and How to Use it? {Variables, Arguments, Return}
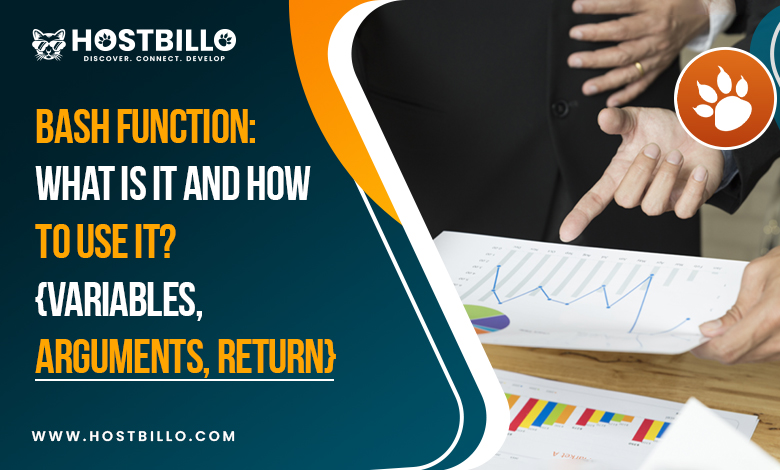
Introduction
Bash functions are an essential part of Bash scripting. They allow you to write reusable code blocks that can be used throughout your scripts. They can help you streamline your workflow, simplify complex tasks, and make your scripts more modular and easier to maintain. In this guide, you will get insights into Bash functions in detail, covering what they are, how to create them, and how to use them effectively. We will also delve into variables, arguments, and return statements, which are essential components of Bash functions. You will also learn some practical examples that can help you master these concepts. So, let’s get started!
Bash Functions: What it is?
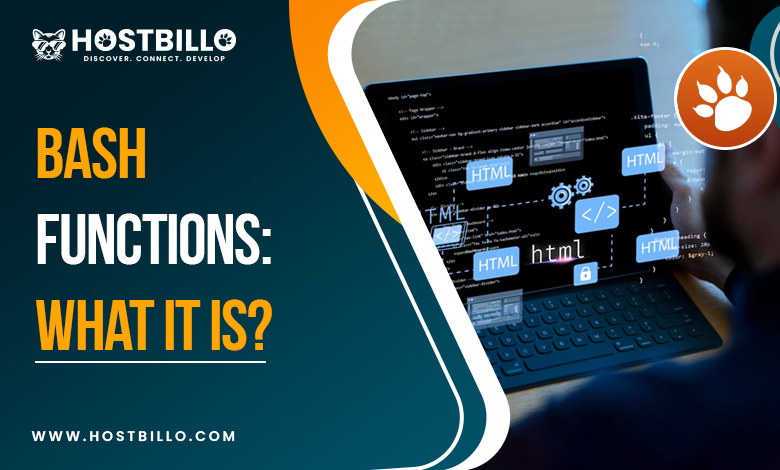
Bash is a Unix shell and command language. It provides a wide range of functionality for interacting with the operating system. One powerful feature of Bash is its ability to define and use functions. These functions are reusable blocks of code that can be called from within a Bash script or interactively in a terminal session.
The bash function can take arguments and can also return values. This makes it a versatile tool for automating tasks and processing data. They can also access and modify environment variables, making it easy to pass data between functions or set configuration values. By learning how to use Bash functions, you can greatly increase your productivity and efficiency when working with the command line.
Steps to Use Bash Functions

Bash Function Syntax
To create a Bash function, use the following syntax:
function function_name {
# Function commands go here
}
You can also use the shorthand syntax:
function_name() {
# Function commands go here
}
Declaring and Calling a Function
To declare a function, use the function keyword or the shorthand syntax shown above, followed by the function name, and then the function commands enclosed in curly braces. For example:
function my_function {
echo “Hello, world!”
}
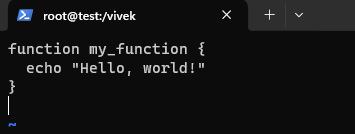
To call the function, simply use its name followed by a set of parentheses:
my_function
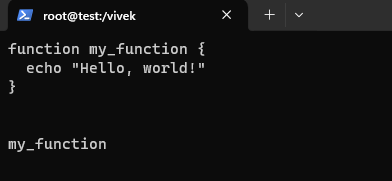
This will execute the commands inside the function.
Output:
Declaring and Calling a Function in the Terminal
You can also declare and call Bash functions directly in the terminal. Simply type the function definition and press Enter, and then call the function by typing its name followed by parentheses. For example:
$ function my_function {
> echo “Hello, world!”
> }

$ my_function
Hello, world!

Where is a Bash Function Defined?
By default, Bash functions are defined in the current shell session and can be accessed from any script or terminal session that runs in the same shell. However, you can also define functions in a script file and then execute that script to make the functions available.
Deleting a Bash Function
To delete a Bash function, use the unset command followed by the function name. For example:
unset my_function

This will remove the my_function function from the current shell session.
Bash Function Variables
In Bash scripting, a function is a set of instructions that can be called multiple times within a script. Variables are an essential part of Bash functions, as they allow data to be stored and manipulated within the function.
Function variables in Bash can be divided into two types: local and global variables. Local variables are defined within a function. They can only be accessed within that function. Global variables, on the other hand, can be accessed throughout the entire script.
Local variables are defined using the ‘local’ keyword followed by the variable name. For example, to create a local variable called ‘var1’ within a function, you would use the following syntax:
function myfunction {
local var1=”Hello World”
}

Once the variable has been defined, it can be manipulated and used within the function as needed.
Global variables, on the other hand, are defined outside of the function and can be accessed throughout the entire script. To create a global variable, simply define it outside of any functions. For example:
#!/bin/bash

myvar=”Global variable”

function myfunction {
echo “The value of myvar is: $myvar”
}

myfunction

In this example, the function ‘myfunction’ is defined to print out the value of the global variable ‘myvar’. When the function is called, it will output “The value of myvar is: Global variable”.
It is important to note that global variables should be used sparingly in Bash function scripts, as they can cause unintended side effects and make debugging more difficult. In general, it is best to use local variables within functions and pass data between functions using arguments and return values.
Bash Function Arguments
In Bash function script can accept arguments, allowing you to pass data into the function for processing. This can be a powerful tool, as it permits you to create reusable code that can handle different sets of data without having to write separate functions for each scenario.
Bash function arguments are passed in as positional parameters, with the first argument being referenced as $1, the second as $2, and so on. For example, the following bash function takes two arguments and concatenates them together:
function concat_strings {
echo “$1$2”
}

concat_strings “Hello” “World”

When this function is called with “Hello” as the first argument and “World” as the second argument, it will output “HelloWorld”.

You can also use the special variables “$@” and “$*” to refer to all of the arguments passed into the function. For example:
function print_args {
for arg in “$@”
do
echo “$arg”
done
}
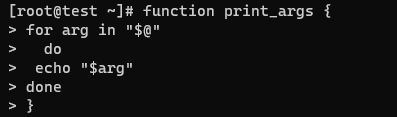
print_args “Hello” “World”

In this example, the function ‘print_args’ takes all of the arguments passed in and iterates over them using a ‘for’ loop. When called with “Hello”, “World”, and “!” as arguments, it will output:
Hello
World
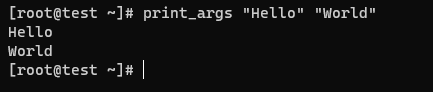
Bash functions can also have default values for arguments, which will be used if the argument is not passed in. For example:
function greet {
name=${1:-“World”}
echo “Hello, $name”
}
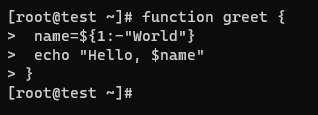
greet

greet “John”
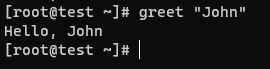
In this example, the ‘greet’ function takes one argument, with a default value of “World” if no argument is passed in. When called without an argument, it will output “Hello, World!”. When called with “John” as the argument, it will output “Hello, John!”.
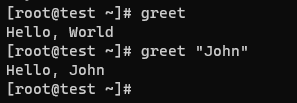
Bash Function Return
In Bash scripting functions can also return values. They allow you to pass data out of the function for further processing or to use in other parts of your script. This can be a powerful tool, as it enables you to create modular and reusable code that can perform specific tasks and return results as needed.
To return a value from a Bash function, you can use the ‘return’ keyword followed by the value to be returned. For example, the following function takes two arguments and returns their sum:
function add_numbers {
sum=$(( $1 + $2 ))
echo $sum
}
result=$(add_numbers 5 10)
echo “The result is $result”
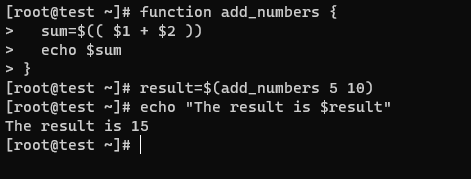
In this example, the ‘add_numbers’ function takes two arguments and adds them together, storing the result in a variable called ‘sum’. The ‘return’ keyword is then used to pass the value of ‘sum’ out of the function, where it is assigned to the variable ‘result’ and printed out using the ‘echo’ command.
You can also use the special variable “$?” to access the return value of a function. For example:
function is_even {
if (( $1 % 2 == 0 ))
then
return 0
else
return 1
fi
}
is_even 4
echo “The return value is: $?”
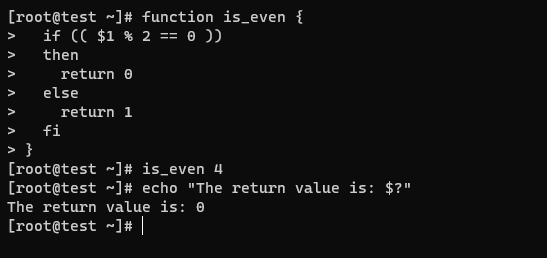
In this example, the ‘is_even’ function takes one argument and checks if it is even or odd. If the number is even, the function returns 0, and if it is odd, it returns 1. The ‘$?’ variable is then used to print out the return value of the function, which will be either 0 or 1 depending on the input.
Conclusion
Bash function arguments are a powerful tool that can help you write cleaner and more efficient scripts in Bash. They enable you to define reusable code blocks that can be called from other parts of your script or directly in the terminal. By using variables, arguments, and return statements, you can create more flexible and dynamic functions that can take input, perform operations, and return output.
In this guide, we’ve covered the syntax for declaring and calling Bash functions, as well as how to declare and call them in the terminal. We’ve also discussed where functions are defined and how to delete them. With these tools and concepts in hand, you can write more robust and maintainable Bash scripts that can help you automate tasks and streamline your workflow.
Also Read: Tally on the Cloud for Accountants: Streamlining Your Workflow