How to Add and Update Items to a Dictionary in Python?
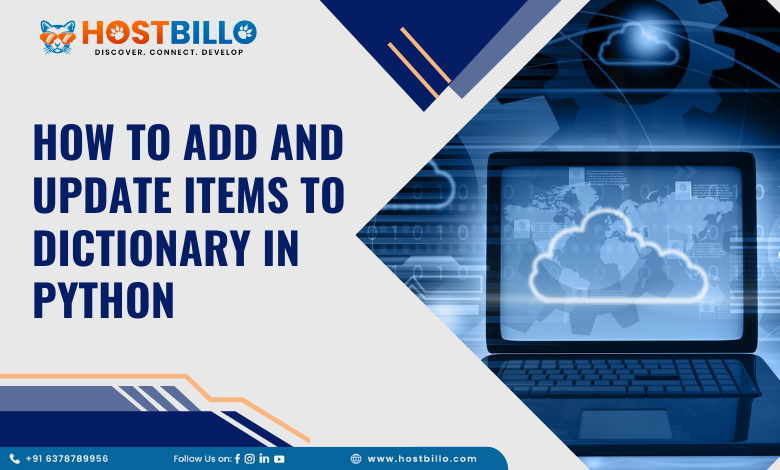
Introduction
Python dictionaries are versatile data structures that allow you to store key-value pairs. Knowing how to add and update items to a dictionary in Python is a fundamental skill for any programmer. With this exhaustive guide, we will discuss numerous methods to readily incorporate new items into your dictionary and update existing ones. It does not matter if you are a newbie or an expert developer, understanding these techniques will enhance your Python programming proficiency.
How to Add Items to a Dictionary in Python
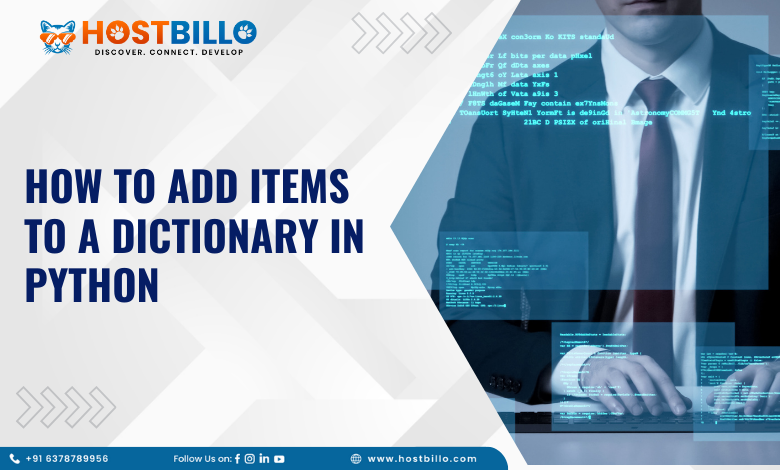
Method 1: Utilizing The Assignment Operator
The assignment operator is the simplest and most direct method to add items to dictionary Python. Employing this approach is particularly intuitive and effective for single-item additions. By leveraging the square bracket notation, you create a new key-value pair, seamlessly expanding your dictionary. This method’s elegance lies in its readability and straightforward syntax, making it ideal for quick insertions in your code. Whether initializing an empty dictionary or enhancing an existing one, the assignment operator provides a concise and clear solution, making your Python code more expressive and manageable.
# Create an empty dictionary
my_dict = {}
# Add an item using the assignment operator
my_dict['key'] = 'value'
Method 2: Employing update()
The update() method is a robust and efficient way to manipulate Python dictionaries, specifically when dealing with multiple items. This method excels in situations where you have a predefined set of key-value pairs to add or update. By passing a dictionary containing the new items as an argument to update(), you can seamlessly merge the contents, ensuring that keys are updated or added without the need for manual iteration. This method not only enhances code readability but also contributes to code maintainability, as it encapsulates the update logic within a single function call, promoting modular and organized code structures.
# Existing dictionary
my_dict = {'key1': 'value1', 'key2': 'value2'}
# New items to add or update
new_items = {'key3': 'value3', 'key4': 'value4'}
# Update the dictionary
my_dict.update(new_items)
Method 3: Using __setitem__
For developers seeking a more specialized and direct approach to add items to the dictionary Python, the __setitem__ method provides a unique avenue. This method permits you to set the value for a specific key directly. While less commonly used, it can be advantageous in situations where a more granular control over the assignment process is required. By invoking __setitem__, you gain a deeper understanding of the dictionary’s inner workings, allowing for a more customized and precise handling of key-value assignments. This method caters to those scenarios where a nuanced approach to dictionary manipulation is paramount.
# Create an empty dictionary
my_dict = {}
# Add an item using __setitem__
my_dict.__setitem__('key', 'value')
Method 4: Utilzing The ** Operator
The ** operator, also known as the unpacking operator, introduces a concise and expressive way to add multiple items to a Python dictionary. This method excels when merging dictionaries, offering a clean and efficient alternative to traditional iteration-based approaches. By leveraging the ** operator, you can merge dictionaries with a single line of code, enhancing code conciseness and readability. This method is particularly valuable when working with a dynamic set of key-value pairs, allowing for flexibility in dictionary composition. The ** operator is a powerful tool for developers seeking a streamlined approach to dictionary manipulation, contributing to code elegance and efficiency.
# Existing dictionary
my_dict = {'key1': 'value1', 'key2': 'value2'}
# New items to add
new_items = {'key3': 'value3', 'key4': 'value4'}
# Use the ** operator to add items
my_dict = {**my_dict, **new_items}
Method 5: Checking If A Key Exists
Before adding a new item to a Python dictionary, it’s prudent to check whether the key already exists. This preventative measure ensures that existing data is not unintentionally overwritten, avoiding potential data loss or errors. By using a conditional check, such as if ‘key’ is not in my_dict, you can determine whether the key is absent in the dictionary, allowing for a controlled addition of new key-value pairs. This method promotes code safety and reliability, especially in situations where data integrity is essential. Implementing this check as a best practice enhances the robustness of your Python code, preventing inadvertent modifications and fostering a more defensive programming approach.
# Existing dictionary
my_dict = {'key1': 'value1', 'key2': 'value2'}
# Add a new item only if the key doesn’t exist
if ‘key3’ not in my_dict:
my_dict['key3'] = 'value3'
Method 6: Using A For Loop
Utilizing a for loop to add or update items in a Python dictionary introduces a dynamic and versatile approach. This method is particularly useful when the update logic is based on specific conditions or computations. By iterating through the keys of the dictionary, you can dynamically adjust values, providing a fine-grained control over the update process. This method’s flexibility allows for the implementation of complex update logic, accommodating diverse scenarios in your Python projects. Whether incrementing values, applying mathematical operations, or implementing conditional updates, the for loop method offers a customizable solution, contributing to code adaptability and maintainability.
# Existing dictionary
my_dict = {'key1': 10, 'key2': 20, 'key3': 30}
# Update values based on a condition
for key in my_dict:
my_dict[key] += 5
Method 7: Using zip
The zip function presents an elegant and concise method for creating and updating Python dictionaries. This method is especially valuable when dealing with separate lists of keys and values. By employing zip, you can seamlessly pair corresponding elements from two sequences, creating key-value pairs for your dictionary. This approach is not only efficient but also enhances code readability, as it succinctly captures the relationship between keys and values. The zip method is a powerful tool for developers seeking a compact and expressive way to initialize or update dictionaries, streamlining the process of dictionary creation based on multiple sequences.
# Existing keys
keys = ['key1', 'key2', 'key3']
# New values
values = ['value1', 'value2', 'value3']
# Create a dictionary using zip
my_dict = dict(zip(keys, values))
How to Update a Python Dictionary
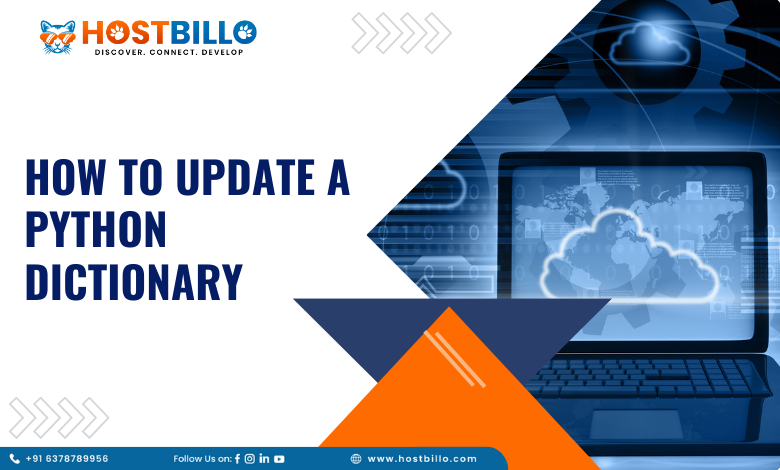
Updating a Python dictionary is a pivotal skill for developers, ensuring data integrity and adaptability in dynamic applications. In this section, we will delve into various techniques and methods to efficiently update existing items and incorporate new ones into your Python dictionaries.
1. Updating Using The Assignment Operator:
The easiest way to update a dictionary is by leveraging the assignment operator. By reassigning a new value to an existing key, you can effortlessly update the corresponding value:
# Existing dictionary
my_dict = {'key1': 'value1', 'key2': 'value2'}
# Update the value for ‘key1’
my_dict['key1'] = 'new_value'
This method is straightforward and intuitive, making it an ideal choice for single-item updates.
2. Using the update() Method:
For more comprehensive updates involving multiple items or even merging dictionaries, the update() method proves to be indispensable:
# Existing dictionary
my_dict = {'key1': 'value1', 'key2': 'value2'}
# New items to update or add
update_items = {'key1': 'updated_value', 'key3': 'value3'}
# Apply the update
my_dict.update(update_items)
The update() method ensures efficient modification and expansion of your dictionary based on predefined sets of key-value pairs.
3. Conditional Updates:
Implementing conditional updates safeguards your dictionary manipulation process, allowing you to modify values based on specific conditions:
# Existing dictionary
my_dict = {'key1': 10, 'key2': 20, 'key3': 30}
# Conditionally update values
for key in my_dict:
if key == 'key2':
my_dict[key] += 5
This approach provides flexibility in updating only specific items, enhancing control over your dictionary’s evolution.
4. Using Dictionary Comprehension:
For a concise and Pythonic approach, dictionary comprehension enables you to update values based on a condition:
# Existing dictionary
my_dict = {'key1': 10, 'key2': 20, 'key3': 30}
# Update values based on a condition
my_dict = {key: value + 5 if key == 'key2' else value for key, value in my_dict.items()}
This method permits for efficient and expressive updates tailored to your specific criteria.
5. Dynamic Updates with Functions:
Implementing custom functions for updating values adds a layer of sophistication to your Python dictionary manipulation:
# Existing dictionary
my_dict = {'key1': 10, 'key2': 20, 'key3': 30}
# Custom update function
def update_value(value):
return value * 2
# Apply function to update values
my_dict = {key: update_value(value) for key, value in my_dict.items()}
This technique facilitates dynamic updates based on your defined logic, promoting modular and reusable code.
Also Read: Check Python Version in Linux, Mac, & Window
Conclusion
In the realm of Python programming, the proficiency to seamlessly add and update items in dictionaries is a cornerstone skill. As we conclude our exploration into these dynamic operations, it becomes evident that the versatility of Python dictionaries lends itself to a myriad of approaches for efficient manipulation. From the simplicity of using the assignment operator for individual additions to the comprehensive nature of the update() method for handling predefined sets, each technique offers a unique perspective on enhancing your Python dictionaries. The choice between methods depends on the specific requirements of your project, whether it’s a single-item addition, a bulk update, or a nuanced adjustment based on conditions.
Remember the significance of preventive measures, such as checking for the existence of a key before adding a new item. This ensures the integrity of your data and guards against unintentional overwrites, contributing to the reliability of your Python codebase. The inclusion of methods like dictionary comprehension and the utilization of functions for dynamic updates further expands your toolkit. These advanced techniques provide not only efficiency but also expressiveness, allowing you to craft code that is both powerful and readable.
As you continue your Python journey, incorporating these methods into your repertoire will undoubtedly bolster your ability to navigate the intricate landscape of dictionary manipulation. Whether you are working as a beginner learning the basics or an expert developer seeking to optimize your code, mastering these operations is paramount. Thus, the art of adding and updating items in Python dictionaries is not just about syntax; it’s about understanding the nuances of each method and selecting the one that aligns best with your specific use case. With these newfound skills, you are well-equipped to navigate the diverse challenges of Python development, creating robust and adaptable solutions for your projects. Happy coding!